WPF .NET Framework : Penerapan MVVM di Windows Presentation Foundation
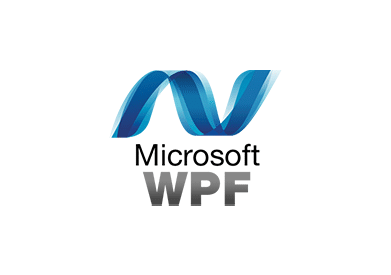
Kali ini saya akan mencoba untuk membuat aplikasi Item Penjualan sederhana menggunakan WPF dengan menerapkan arsitektur MVVM (Model View View Model). MVVM sendiri bisa dikatakan juga merupakan MVC (Model View Controller) yang memisahkan antara logic dengan GUI (Graphical User Interface). Untuk implementasinya sebagai berikut.
- Membuat Project WPF.
- Membuat Model
- Melakukan desain GUI (view)
Untuk hasilnya akan membentuk window yang kira-kira seperti gambar dibawah ini.
- Membuat ViewModel
- Melakukan Data Binding
Untuk menghubungkan antara model, view, dan view model perlu didefinisikan sebuah DataContext. Untuk implementasinya adalah dengan membuat MainWindow.xaml.cs sebagai berikut.
Kemudian kita lakukan Data Binding dengan mengubah MainWindow.xaml menjadi sebagai berikut.
Hasil dari aplikasi yang telah kita buat hingga saat ini menjadi sebagai berikut.
Komentar
Posting Komentar